Mesh example: More geometryΒΆ
This example is from from Calfem for Pyhon Mesh Manual (Mesh_Ex_02.py)
Creating geometry from B-Splines and circle arcs. Also shows how to set ID numbers for geometry entities and how to specify element density.
[2]:
import numpy as np
import calfem.geometry as cfg
import calfem.mesh as cfm
import calfem.vis_mpl as cfv
Define geometry
All mesh generation starts by creating a Geometry object.
[3]:
g = cfg.Geometry()
Add points
In this example we set the IDs manually.
[4]:
g.point([-2, 0], ID=0)
elSize determines the size of the elements near this point.
[5]:
g.point([0, 1], ID=1, el_size=5)
elSize is 1 by default. Larger number means less dense mesh. Size means the length of the sides of the elements.
[6]:
g.point([1, 0], 2, el_size=5)
g.point([0, -2], 3)
g.point([0, 0], 4, el_size=5)
g.point([.5, .2], 5)
g.point([-.5, .5], 6)
g.point([-.7, -.5], 7)
Add curves
The 3 points that define the circle arc are [start, center, end]. The arc must be smaller than Pi.
[7]:
g.circle([1, 4, 2], 2)
BSplines are similar to Splines, but do not necessarily pass through the control points.
[8]:
g.bspline([5, 6, 7, 5], 5)
g.bspline([1, 0, 3, 2], 4)
Add surfaces
[9]:
g.surface([4, 2], [[5]])
Markers do not have to be set when the curve is created. It can be done afterwards. Set marker=80 for curves 2 and 4:
[10]:
for curveID in [2, 4]:
g.curve_marker(curveID, 80)
Generate mesh
Create a mesh object and set its attributes.
[11]:
mesh = cfm.GmshMesh(g)
mesh.el_type = 3
mesh.dofs_per_node = 2
mesh.el_size_factor = 0.05
Create the finite element mesh using the create() method of the mesh object.
[12]:
coords, edof, dofs, bdofs, elementmarkers = mesh.create()
Info : GMSH -> Python-module
Visualise mesh
Draw geometry
[13]:
%matplotlib inline
# %matplotlib widget
[14]:
cfv.figure(fig_size=(8,8))
cfv.draw_geometry(
g,
label_curves=True,
title="Example 2 - Geometry"
)
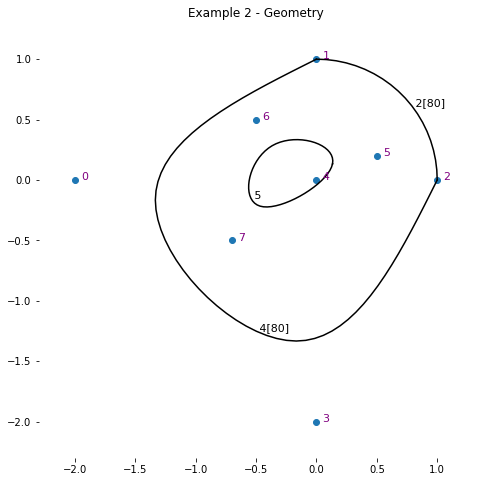
Draw mesh
[15]:
cfv.figure(fig_size=(8,8))
cfv.draw_mesh(
coords=coords,
edof=edof,
dofs_per_node=mesh.dofs_per_node,
el_type=mesh.el_type,
filled=True,
title="Example 2 - Mesh"
)
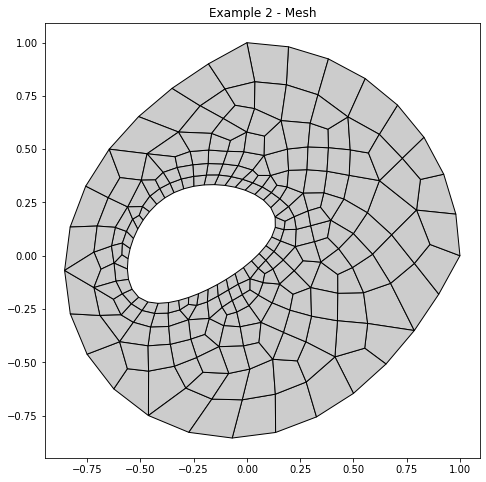